Password Reset Flow
The Password Reset Flow runs during the password reset process when a user completes the first challenge, typically a link to the user's email, but before a new password is set. You can use this flow to challenge a user with an additional multi-factor authentication (MFA) factor or to redirect the user to an external site, such as a third-party verifier.
After verification, users can provide the new password for their account.
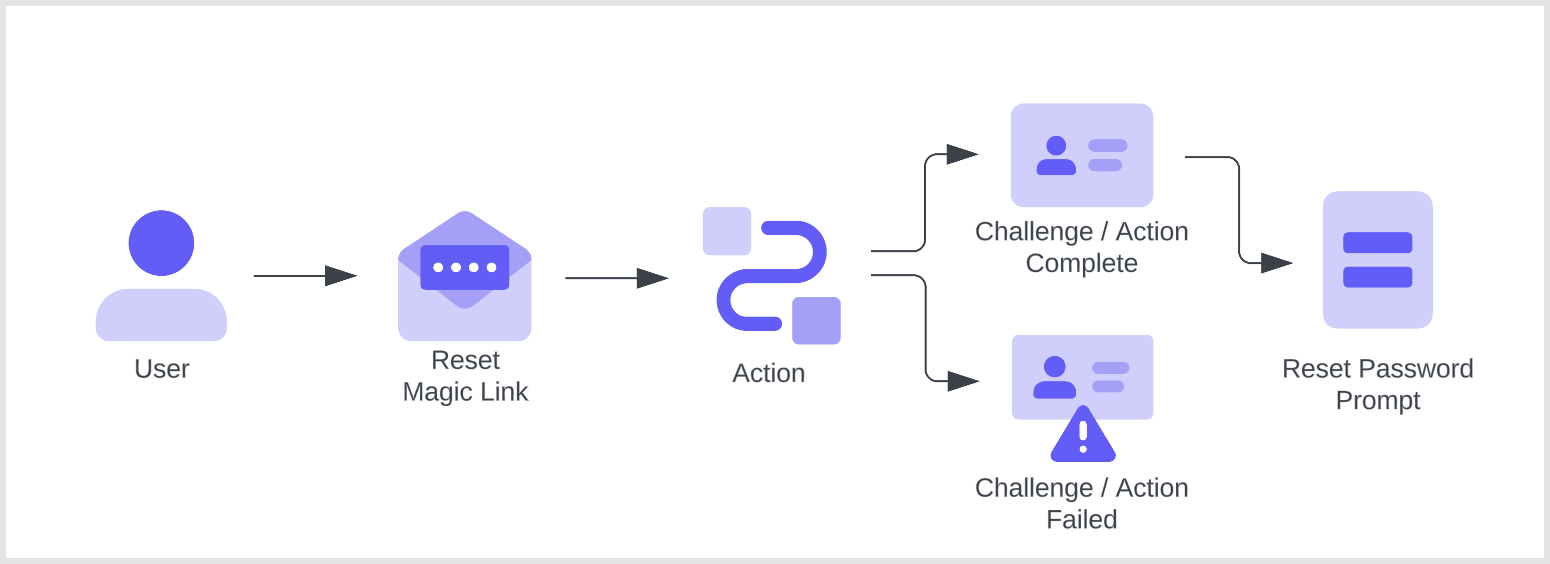
Actions in this flow are blocking (synchronous), which means they execute as part of a trigger's process and will prevent the rest of the Auth0 pipeline from running until the Action is complete.
Triggers
PostChallenge
The post-challenge
trigger is a function that executes after a user completes the first password reset challenge, typically an email magic link. You can create up to four Actions in your tenant that leverage the post-challenge
trigger.
References
Event object: Provides contextual information about a single user logging in via Auth0.
API object: Provides methods for changing the behavior of the flow.
Limitations
The Password Reset Flow does not support Active Directory/LDAP connections.
Common use cases
Secure password reset with additional MFA factors
A password-reset
/ post-challenge
Actions can issue an MFA challenge after the user completes the first challenge. For example, you can issue a WebAuthn-based challenge as a secondary factor if your tenant has WebAuthN enabled as a factor.
/**@type {PasswordResetPostChallengeAction}**/
module.exports.onExecutePostChallenge = async (event, api) => {
const enrolledFactors = event.user.enrolledFactors.map((x) => ({
type: x.type
}));
api.authentication.challengeWith({ type: 'webauthn-roaming' }, { additionalFactors: enrolledFactors });
};
Was this helpful?
Redirect users to a third-party application
In addition to an MFA challenge, you can also try adding a redirect in the custom Action, for example, to a third-party verifier or risk assessor.
/** @type {PasswordResetPostChallengeAction}
* This sample action redirects the user to an example app
* and then continues the action after the redirect to challenge
* the user with an MFA factor
*/
module.exports.onExecutePostChallenge = async (event, api) => {
// Send the user to https://my-app.example.com
api.redirect.sendUserTo('https://my-app.example.com');
};
module.exports.onContinuePostChallenge = async (event, api) => {
const enrolledFactors = event.user.enrolledFactors.map((x) => ({
type: x.type
}));
// Challenge the user with email otp OR another enrolled factor
api.authentication.challengeWith({ type: 'email' }, { additionalFactors: enrolledFactors });
// Example of how to challenge the user with multiple options
// in this case email otp OR sms otp
// api.authentication.challengeWithAny([{ type: 'email' }, { type: 'sms' }]);
};
Was this helpful?
The Actions pipeline is not active while Auth0 redirects the user. Once the user continues the Auth0 login process, the Actions pipeline resumes. Actions that were executed prior to the redirect are not executed again. To learn more, review Redirect with Actions.